In this lesson you will create 3 separate web pages that showcase pictures from a trip to Costa Rica and learn about using div tags in conjunction with CSS to position content. When you are finished you will link the three files together into sort of a mini website. Let’s get started.
- Download div_layout.zip. Unzip it and drag the div_layout folder into your work folder.
- Create a new web page and save it inside the div_layout folder as monkeys.html.
- Create a new CSS file named div_layout.css and save it into the div_layout folder.
- Attach div_layout.css to monkeys.html (by now you should know how to do this).
- Create a new style called #all_content inside of div_layout.css. This style, as its name suggests, will define the div tag that contains all of your content.
- Be sure and add the # sign to the style name. The purpose of adding # to the style name is to create an id style to be used only one time per document.
- Add the following characteristics to #all_content (remember the semicolons):
- width:1000px; height: 2200px;
- margin-left: auto; margin-right: auto;
This will center all content. - background-color: red; (or some other color! You can change this later, for now it is useful to be able to see our div tag and the color doesn’t matter much).
- Now that you have made this style it is time to apply it to a div tag and find out what it does. Immediately after the tag enter <div id=”all_content”></div>. (replace the curly quotes with straight quotes if you copy this).
- The part that says <div id=”all_content”> is the starting point for the div tag
- </div> is the ending point of the div tag.
- Preview your document. If everything worked properly you should have a 1000 pixel wide red blob in the middle of your browser’s window.
- You are about to insert a series of div tags inside of the all_content div tag. This process can be confusing when you do it for the first time. In order to make it less confusing we are going to insert a ‘comment’ immediately after the tag that closes the all_content div tag: </div>. Comments are invisible to the browser but can be a very useful feature for web developers. When you finish, your code should look something like:
<div id=”all_content”> </div><!–all_content ends here–> - Now create a new ID style in div_layout.css, just as you did with #all_content named #banner. Set your background color to whatever you like, height to 92 pixels, width to 100%, and margin-top to auto. Give it a background color as well but something other than red.
- Insert a new div tag between <div id=”all_content”> and </div> and apply the banner style to the new div tag. Your source code should look more or less like this:
<div id=”all_content”> <div id=”banner”></div> </div><!–all_content ends here–> - Preview in your browser. The banner should appear at top of your page.
- Create another new ID called #leftlinks with a width of 150px, height of 2100px and
- Float it to the left. (float: left;)
- Give it a background color other than red so that you will be able to see it.
- Insert a div tag after the banner and attach leftlinks to it <div id=”leftlinks”></div> .
- Create an ID style called #right_side_content. Set the width to 840px, height to 2100px, and float it left as well.
- Give this rule yet another background color that is different from the other two background colors that you have used.
- Insert a div tag after the leftlinks div tag and attach right_side_content to it.
<div id=”right_side_content”></div>
- Type a title into your banner. Now return to your css file and put in some initial text values for your banner such as font-size, font color ( color: #000000; for example). Also add a font family ( enter font-family: and your editor should prompt you with some choices). Once you apply the changes, the look of the text within your banner should change. If you don’t like it, modify the #banner rule until you are content. While you are at it set the display to block (display:block;) and experiment with the letter spacing ( letter-spacing: 6px; for example), vertical alignment (enter vertical-align: and look for prompts) and any other values that you want to play with. For inspiration, you may want to visit w3school’s font information page: http://www.w3schools.com/cssref/pr_font_font.asp
- Create a Css tag named body and set a background color. This should change the background for the entire page!
- Take a look at your page does everything appear to be in its proper place? If not, fix it. Otherwise, move on.
Repurpose your work!
An important trick for moving quickly in web development is to repurpose work that already exists. You just invested time developing monkeys.html so let’s leverage that investment by using monkeys.html to create the two additional webpages that we will need for this exercise. Once all three webpages exist, we will develop them further.
- Save monkey.html again, but this time save as and create a brand new file called birds_and_bananas.html. Save as again and create another file named waterfalls.html. At this point you should have 3 identical files open, but with separate names for each. Your next task is to add content to each of those files.
Adding and Positioning Content
Let’s start with birdsandbananas.html. We are going to start by inserting an image named birdsonbananas.jpg (inside of the pix folder) inside of the right_side_content div tag. Before we do that, however, we also want to create a special div tag that is tailored just for the birdsonbananas.jpg image. So we need to know the size of that image.
There are several ways to get the dimensions of an image. The screen capture below shows one that works well with the Macintosh OS by viewing folders and files with the ‘columns’ view
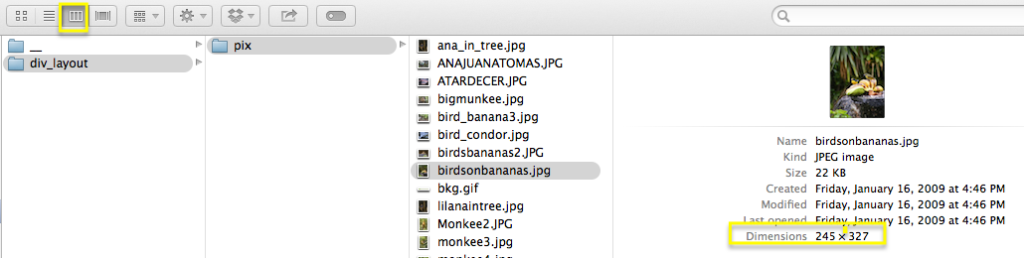
As you can see, the image named birdsonbananas.jpg has a 245px width and a 327px height. Another easy way to find the dimensions of an image is simply to right-click on it and select “Get Info” from the drop down list that appears. Of course, it is also possible to open the image in an image editor such as preview, fireworks, or photoshop and find the dimensions there.
- However you determine dimensions, once you have them create a new class inside of div_layout.css named .bb1 with width:245px; and height:357px; ; as shown below:
.bb1 {width:245px; height:357px; }
You may have noticed that the height of the css rule, .bb1, is greater than the height of the image. That’s because we want to leave a bit of room inside of the div for descriptive text.
- Now insert a div inside of right_side_content, apply your new .bb1 rule to it, and insert birdsonbananas.jpg into the new div as shown below.
<div class="bb1"><img src="pix/birdsonbananas.jpg" alt="birds on bananas"/> </div>
Notice that <img src, in addition to a path to birdsonbananas.jpg, also has an alt tag that describes the image. You learned about alt tags earlier but don’t neglect to use them whenever you insert an image.
- Preview your page. You should see your image but at this point the div tag is invisible. To provide a visual aid that allows you to actually look at your div tag, return to the css file and add a new rule as follows:
#right_side_content div { border: 1px solid #1394ce;
float: left;
margin-left: 5px;
margin-top: 5px;}
This will add a one pixel wide blue border to every div tag that is placed inside of right_side_content, which you may want to remove later so that all div tags don’t have a border, but it’s useful at this stage.
This rule also floats all div tags inside of right_side_content to the left so that they show up side by side instead of on top of each other and adds left and top margins of 5px;- The beauty of defining all of the div tags in right_side_content at once is so that you will not have to add margins and floats to all subsequent classes that you create for the divs that hold your images.
- The beauty of defining all of the div tags in right_side_content at once is so that you will not have to add margins and floats to all subsequent classes that you create for the divs that hold your images.
- Add some descriptive text above or below the picture. If you need additional room for text, expand the size of the div tag by adding more height to the CSS rule that defines it.
- Repeat the same steps that you used with the previous image for a brand new one. If you get lucky and find an image that is the same size as another image that you already added, you can apply a pre-existing class to it.
- Add a few more bird and/or banana images, following the same steps.
- Save the file.Open monkeys.html. Insert the four monkey pictures, following the same steps (div tags, css, etc.) that you did with birds_and_bananas.html.
- .Open waterfall.html and do the same thing.
- Starting with waterfall.html, create links inside of the leftlinks div tag to the monkeys.html, birds_and_bananas.html.
- While you are at it, create an unordered list (ie. bullets, as shown below).
<div id="leftlinks"> <ul> <li><a href="monkeys.html">Monkeys</a> <li><a href="birds_and_bananas.html">Birds and Bananas</a> </ul> </div>
Save waterfall.html and test the links. If they work properly, link the remaining two files to each other and to waterfall.html as well. Test thoroughly. If you have done everything right, you should have a fully functioning website of 3 pages that all connect to each other. If not, ask your instructor for help.