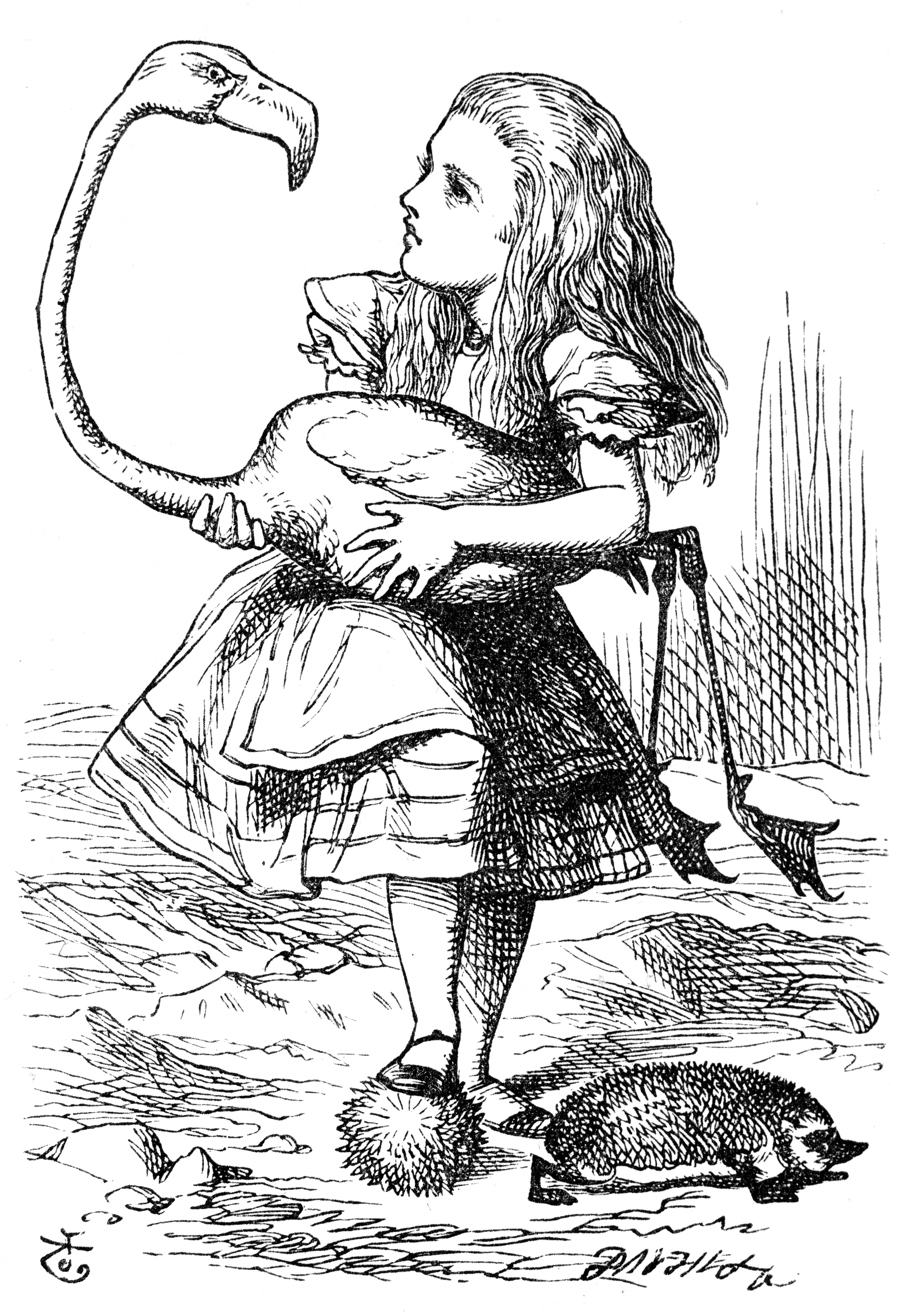
Boolean Values
Simply put, a boolean is another data type, just like a string, array, or number. Unlike other data types, a boolean can only have two values: true
or false
. 1
It can be helpful to think of a boolean as a toggle, or an on-off switch. When the toggle is in the “on” position, the value is true. When the toggle is in the “off” position the value is false.
Here’s an example of a boolean value in a function:
function offWithHisHead() { return false; }
Conditional Logic: If Statements
We’ll see boolean values come into play when we use if
statements. In this context, if
is a keyword that controls the flow of the program using booleans. This is the syntax of an if
statement:
if (condition) { code block; } ... next bit of code...
Here’s how we would interpret that if
statement: “If the condition is true, then execute the code block.” On the other hand, if the condition were false, the code block wouldn’t be executed, and the program would move on to execute the next bit of code.
This might feel a little abstract right now, but as you move through the Free Code Camp exercises in this section, you’ll get a lot of practice with if
statements, and they should make a lot more sense!
Comparison Operators
JavaScript gives us a whole set of operators that allow us to compare two numerical values. These operators will always return a boolean true/false value. This sounds a little complicated, but we basically asking simple questions like: “is a
greater than b
” or “is c
equal to d
.” We’re just asking those questions with JavaScript!
One thing to be aware of here, is that some of the operators will actually convert the data type for you, so that the number 1
would be read the same as the string "1"
. When this happens, it’s called type coercion. You’ll see what we mean in a second.
Equality operator ==
: compares two values and returns true or false based on its findings. It will convert data types for you, so 1
and "1"
will be read as the same value.2
Strict equality operator ===
: This is similar to the equality operator, but it will not convert the data type for you, so if one value is the number 1
and the other is the string "1"
they will not be considered equal.
Inequality operator !=
: This is simply the opposite of the equality operator and will return the value “true” when two values are not equal. The inequality operator will convert data types.
Strict inequality operator !==
: Chances are good that you see where we are going here! The strict inequality operator returns a value of true when two values are not equal. It does not convert the data type for you.
Let’s take another look.
a | b | a == b | a === b | a != b | a !== b |
2 | 3 | false | false | true | true |
4 | "4" | true | false | false | true |
4 | 4 | true | true | false | false |
Type coercion comes into play when a = 4
and b = "4"
. The equality operator and inequality operator treat those two values as the same data type, but the strict equality and strict inequality operators do not.
Why do we need comparison operators? They are incredibly useful when we’ll try to manipulate more advanced data structures down the line. They are also really handy in if
statements. Here’s an example:
function testComparison(val) { if (val == 3) { return "Equal"; } return "Not Equal"; } testComparison(3);
What we’re seeing in the example above is a function
called testComparison
with one parameter: val
. The statement inside the function reads: if val
is equal to the number 3
then return the string "Equal,"
otherwise, return the string "Not Equal."
Okay, let’s tackle some other comparison operators. These should look pretty familiar, so we’ll move through them quickly.
Operator | Interpretation |
a < b | true when a is less than b |
a > b | true when a is greater than b |
a <= b | true when a is less than or equal to b |
a >= b | true when a is greater than or equal to b |
It’s helpful to note that all four of these operators will convert data types for you. We can use these operators in a function just like the other comparison operators. Here’s an example:
function perspective(glass) { if (glass < 50) { return "Half Empty"; } return "Half Full"; } perspective(70);
What will happen when we pass the argument 70?3
The Logical And (&&
) and Logical Or (||
)Operators
Let’s take it a step further. Say we wanted to compare two different boolean values at the same time. The logical and operator will allow us to do that. It will return true
if both operands are true.
The logical and operator is displayed as two ampersands: &&
. Here’s an example of it in action:
if (a > 10 && a < 100) { return "Correct"; } return "Incorrect";
What we’re saying there is “if a
is greater than 10 and less than 100 return “Correct,” otherwise, return “Incorrect.” In other words, both the statement to the left and right of the &&
operator must be true for the boolean value to equal true.
The logical or operator also allows us to compare two different boolean values at the same time, but it will return true
if either statement is true. The logical or operator is made of two pipe symbols: ||
.4 Here’s how it works in an if
statement.
if (b < 10 || b > 100) { return "Correct"; } return "Incorrect";
Here we will return “Correct” if b
is less than 10 or if b
is greater than 100. Simple enough, right?
Else Statements
At this point, we’ve seen how if
statements work: in the examples above, when the if statement is true, we execute the code following the statement. An else
statement works with the if
statement and allows us to move on to execute the next bit of code when an if
statement is false. Basically we’re saying “if this is true do this thing, or else, do this next thing.” Here’s the syntax of an else
statement:
if (condition) { code block; } else { new code block; }
We can easily chain together multiple if
statements by using else if
statements. Here’s what that would look like:
if (height > 100) { return "Alice ate something"; } else if (height < 5) { return "Alice drank something"; } else { return "Down the rabbit hole again"; }
You’re not limited in the number of else if
statements you use, so as you can imagine, your code can get quite lengthy and complex. It’s important to remember that the function is executed from top to bottom, so the order of the statements can matter quite a bit.
Switch Statements
Switch statements are similar to if
statements, in fact, they can can be used to simplify complex else if
statements in certain instances. A switch statement looks for the value of variable among several options (or cases); it uses break
statement to tell JavaScript to stop looking for matches among the cases. Let’s look at an example:
switch(character) { case "tweedle dee": case "tweedle dum": danger = "low"; break; case "MaddHatter": danger = "medium"; break; case "Queen of Hearts": danger = "high"; }
This switch
statement switches on the value of the variable character
. By that we mean, that it compares the value of character
to the values of the cases. If the value of character
is equal to any one of the cases then any code for any case written below the matching case will execute until either a break statement occurs or there are no more cases.
In the above example, if the character
is set to either "tweedle dee"
or "tweedle dum"
then the danger
will be set to "low"
. After which, the switch
breaks when it reaches the break
statement. On the other hand, if we set character
to "Queen of Hearts,"
the danger will be "high"
. Note that you do not need a break
statement after the last case.
You can add a default
statement after the last case
which will be executed if the value doesn’t match any of the cases. Without a default
it is possible that none of the cases match the variable we are switching on. For example, if character
is “Cheshire Cat” in the example above, it will not match any of the cases and we wouldn’t update our danger
result. Here’s what the default statement would look like:
switch(character) { case "tweedle dee": case "tweedle dum": danger = "low"; break; case "MaddHatter": danger = "medium"; break; case "Queen of Hearts": danger = "high"; break; default: danger = "unknown" }
Return Statements
This might seem kind of tacked on and easy to overlook in the Free Code Camp lessons, but it’s a really important (and simple!) concept. A return statement is similar to a break statement. While a break statement ends a switch statement, a return statement ends any function.
A function can actually have multiple return statements, which can be useful when you want to limit further code execution to scenarios where it makes sense. For instance consider this function:
reciprocater(y) { if (y == 0) { return 0 } return 1/y }
This function takes in a value y
and returns it reciprocal 1/y
, however, if y
is 0
, then 1/y
is undefined. The early return
statement ensures that we do not try to divide 1
by 0
.
Operator Quick Reference
Here’s a quick reference of comparison operators that we looked at in this group of Free Code Camp lessons:
Operator | Interpretation |
== | The equality operator is true when values are the same regardless of data type |
=== | The strict equality operator is true when the values AND data type are the same |
!= | The inequality operator is true when two values are not equal regardless of data type |
!== | The strict inequality operator is true when the values and/or data type is not the same |
a < b | True when a is less than b |
a > b | True when a is greater than b |
a <= b | True when a is less than or equal to b |
a >= b | True when a is greater than or equal to b |
&& | The logical and operator is true when the operands to the left and right of it are true |
|| | The logical or operator is true when the operands to the left or right of it is true |
Be aware that boolean values don’t have quotes around them: so it’s true, not “true.” If quotes are present, it will be read as a string value and not a boolean value.↩
Don’t get this confused with the assignment operator (
=
) which is used to assign a value to a variable as invar = 7
.↩Our glass will be “Half Full”, optimism for the win!↩
hold shift and backslash on most keyboards↩