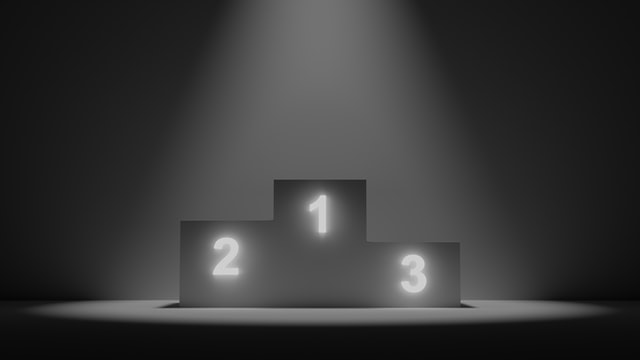
Welcome to your last section of Free Code Camp! You’re almost to the finish line. Let’s finish strong! ???
jQuery
Out of all the JavaScript libraries, one of the most popular and widely used is jQuery. jQuery takes complicated JavaScript tasks and makes them easy, by taking several lines of code and simplifying them into one line—kind of like a shortcut. jQuery uses a less complicated syntax, as expressed in its tagline: write less, do more.
If that sounds a little bit revolutionary to you now, it certainly was back in the mid-2000’s. Not to sound dramatic, but jQuery’s simplification is kinda the best thing ever.
Just like we learned with Bootstrap (the most popular HTML and CSS library), libraries consist of pre-written code for the purpose of quick and easy development.
According to W3Schools, here’s a glimpse at a few of the major web companies that use jQuery:
- Microsoft
- IBM
- Netflix
To summarize, jQuery is a JavaScript file you include in your webpages. You use jQuery to find elements using CSS-style selectors, and then you make these elements do tasks, such as manipulating elements, creating animations, and handling input events.
The jQuery function $()
The function jQuery()
lets you find one or more elements on your page. This is shortened into $()
. This dollar sign ($
) syntax is the most recognizable character of jQuery. 1
The script element
<script> JavaScript here </script>
The <script> element signals to the browser that the code in between the elements is JavaScript. Just like with internal style tags, you can write script in your HTML files, but the more professional way is to create a separate .js file and link it to your HTML file—just like you would an external stylesheet. Here’s how you would link a file called script.js to your HTML page:
<script src="js/script.js"></script>
Typically, just like with CSS, you keep your JavaScript files in their own folder.
To use jQuery, you also have to link to jQuery in your <head>
section. Notice that like with Bootstrap, there’s different iterations of jQuery. Here’s an example of what the jQuery link would look like:
<head>
<script src="jquery-3.4.1.min.js"></script>
</head>
You don’t necessarily need to know this to complete your FreeCodeCamp exercises, but someday you’ll come across jQuery in the wild.
Document ready
Just like you don’t want to pull out a cake from the oven until it’s cooked all the way through, JavaScript doesn’t need to manipulate a page until it’s “ready”. The code you put in $(document).ready(function()
will not run until JavaScript code is ready to execute. If you were to run JavaScript before a page was ready, you could encounter bugs. ?
Don’t forget to end your document ready function: });
Writing jQuery statements
jQuery functions—which belong inside the document ready function— start with the dollar sign operator $
, such as:
$("button").addClass("animated bounce");
jQuery selectors $()
allow you to select and manipulate HTML elements.
You can “find” (or select) HTML elements based on their name, id, classes, types, attributes, values of attributes and much more.
In the above example, you’re selecting your button
class (from your HTML) and you’re using jQuery to add the bounce
class to all of of your button
elements.
Here’s what a jQuery function is saying in layman’s terms:
$(some element).manipulate with("some effect");
Remember from your HTML and CSS module, a class selector uses periods (classes meet during school periods), and ID selectors use hashtags (every person has an individual phone number). You can target elements with either one:
To target the class well
: $(".well)
To target the id target3
: $("#target3")
And of course, you can target HTML elements based on name, attributes, and much more.
Dynamically change CSS with jQuery
jQuery has a function called .css()
that allows you to change the CSS of an element.
Here’s how we would change the target1
color to blue:
$("#target1").css("color", "blue");
Why would someone decide to make style changes this way?
Let’s say you wanted to make your style more ~dynamic~. You could have certain colors change when a user selects something, highlight some functionality, or emphasize a feature. JQuery can be used to dynamically alter and tweak CSS.
Change HTML elements with jQuery
Using .html()
, you can change the content in between the start and end tag of an HTML element. You can change the HTML markup.
Let’s say you just wrote a ton of copy all about your company’s “Spring Sale,” but executives decide last-minute to call it a “Coronavirus Blowout” instead. You could use jQuery functions to quickly modify your content.
Function chaining ⛓
Something that makes jQuery so popular is its ability to chain multiple functions together elegantly, without lines and lines of spaghetti noodle code mess. The ability to stick jQuery functions together is called function chaining.
In the FCC example, Cloning Element Using jQuery, you encounter function chaining for the first time:
$("#target2").clone().appendTo("#right-well");
To put this in human-speak:
$(some element).manipulate with (“first effect”).manipulate with (“second effect”)
These jQuery lessons may be short and sweet, but that’s the nature of jQuery. By now, you’ve learned some of the advantages of jQuery and how to write jQuery functions.
Ready to expand your knowledge of jQuery? Check out these resources:
And that’s it!
That wraps up your Free Code Camp journey for this course! Congrats!
Some developers call the dollar sign operator bling✨. ↩