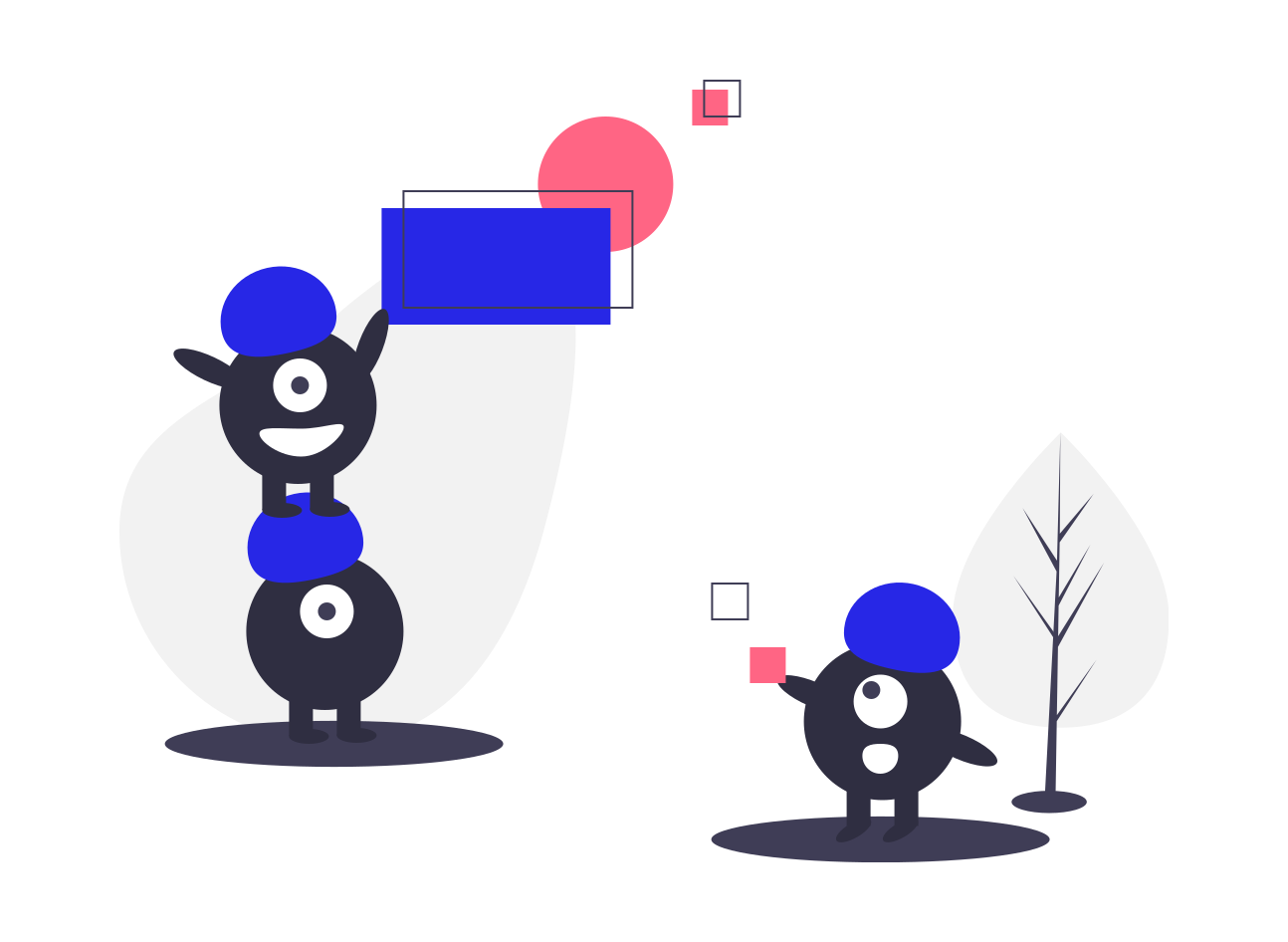
Objects
The lessons in FCC-20 are all about objects.
“What’s an object?” you might reasonably ask. FreeCodeCamp tells us that “objects are similar to arrays, except that instead of using indexes to access and modify their data, you access the data in objects through what are called properties. Objects are useful for storing data in a structured way, and can represent real world objects, like a cat.” FCC goes on to provide a sample cat object:
var cat = {
"name": "Whiskers",
"legs": 4,
"tails": 1,
"enemies": ["Water", "Dogs"]
};
This sample cat
object has the properties name
, legs
, tails
, and enemies
.
Another definition of an object, from Eloquent JavaScript, states that “object[s] are arbitrary collections of properties.”1However, both definitions beg the essential question: what even is a property?
Two pieces of good news:
- Properties are actually fairly straightforward!
- We’ve already met them!
For our purposes, you can think of a property as a thing that you can access from a piece of data (such as a variable, a constant, etc.). That’s it!
When have we already met properties? All the way back when we were trying to find the length of a string. .length
is a property of the string data type that gives us the length of that string. All of the bracket notation we learned for strings and arrays? Properties! Hopefully, that helps you feel a bit more familiar with accessing them in our present context of working with objects, either through dot notation (object.property
) or bracket notation (object["property name"]
).
The next several FCC lessons help you get a sense of all the things you can do with objects—access their properties with variables, as well as update, add, and delete their properties, too.
The ensuing lessons then show you a few more sophisticated things you can do with objects, such as using them for lookups and manipulating complex objects, including how to access nested objects and nested arrays. All of this builds to the Record Collection lesson, which, in its own challenging way, shows you the power of objects as rich data structures and also fully introduces us to JSON (JavaScript Object Notation).
Hang in there with the Record Collection lesson. It’s challenging, but doable. And, learning to work with JSON objects (in conjunction with jQuery, which you’ll learn in a bit) is one of the most practical things you’ll learn from the JavaScript unit. Why? Many of the web’s most popular services (Facebook and Twitter, among many others) provide access to their data via JSON objects.
To conclude, one bit of information that isn’t necessary to complete your FreeCodeCamp work, but that’s fairly essential to know to have an informed conversation about JavaScript objects: objects can also contain functions (which are called methods, in this context). If you’re curious about how this works or why it’s important, you can go read more at the aforementioned (and excellent!) Eloquent JavaScript, but be warned: if you read even just a little over at that site, you’ll likely find yourself wanting to read much more.
This definition’s validity is shown by the fact that after its introductory lesson on objects, FCC’s next two lessons are about accessing an object’s properties.↩