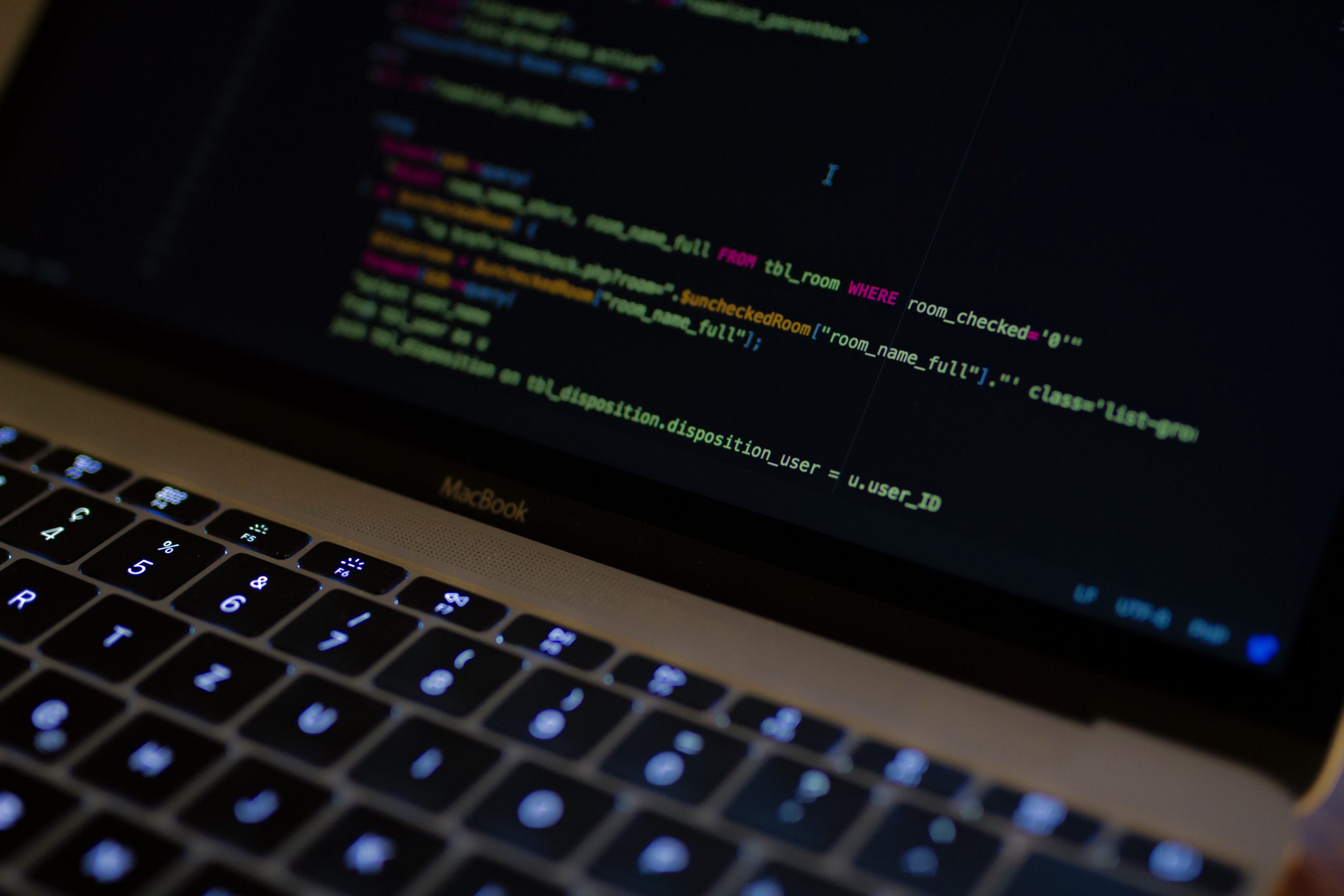
Open a new HTML file in Visual Studio Code and save it as test.html in your Dropbox in a folder called playground or WebDev Test Files (or something similar, this is a just a place for practice!)
Copy and paste the HTML boilerplate or let VSC autofill the boilerplate for you by typing html and then selecting html:5
from the dropdown options.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> </body> </html>
Within the <body></body>
tags, copy and paste the following code:
<body> <h1>Main Heading</h1> <h2>Subheading</h2> <h3>Another Slightly Smaller Subheading</h3> <p>This is the paragraph section</p> </body>
Save your work (click ⌘+s if you’re on a Mac or Control+S if you’re on a Windows machine). Once it’s saved, right click on the test.html file that you just created and click “Open with Chrome” to preview your work.1 Hey, you made a webpage! Pretty cool, right? It should look like what you see below.
Main Heading
Subheading
Another Slightly Smaller Subheading
This is the paragraph section.
HTML Elements
HTML elements are how developers structure the content and information on websites. You’ve seen them before in brackets: < >
. Everything inside those brackets is called an HTML element.
For instance, <body></body>
is an HTML element. Elements are often, but not always, made up of an opening tag (<body>
) and a closing tag (</body
).
Can you tell the difference between the opening and closing tag? The forward slash, /
, indicates the closing tag. HTML elements tell the browser how to display the content placed in between the tags.
For instance, <h1>
is telling the browser this is a main heading. The browser knows everything from <h1>
to </h1>
is main heading. <h2>
is telling the browser the words from <h2>
to </h2>
should be displayed as a subheading. And so on and so forth!
You can think of tags as boxes. The opening tag (<p>
) is a box, and the closing tag (</p>
) is the box lid. Everything inside the box is what you specify it to be.
Those letters in the tags (h1, h2, p, etc) aren’t random. These characters are part of the HTML syntax and express the tag’s purpose.
Hold up! What even is HTML?
HTML files control the structure of webpages.
HTML5, HyperText Markup Language, is the standard markup language of the web. These HTML tags are called “markup.” HyperText is describing the way that HTML files use links to quickly jump around.
Free Code Camp also offers a description of HTML:
The HyperText part of HTML comes from the early days of the web and its original use case. Pages usually contained static documents that contained references to other documents. These references contained hypertext links used by the browser to navigate to the reference document so the user could read the reference document without having to manually search for it.
As web pages and web applications grow more complex, the W3 Consortium updates the HTML specification to ensure that a webpage can be shown reliably on any browser. The latest version of HTML is HTML5.
For more background info about HTML, check out this article.
White space
White space can make your code cleaner and easier to read. Your browser will not display this white space, so feel free to indent or add spaces to your heart’s content.
Fun tip: If your browser comes across two spaces next to each other, it only displays one space.
For example:
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello world!</title> </head> <body> <h1>Main Heading</h1> <h2>Subheading</h2> <h3>Another Slightly Smaller Subheading</h3> <p>This is the paragraph section</p> </body> </html>
Displays the same as:
<body> <h1>Main Heading</h1> <h2>Subheading </h2> <h3>Another Slightly Smaller Subheading</h3> <p>This is the paragraph section</p> </body>
That being said, please don’t format your HTML the second way—that’s just to illustrate the point that white space doesn’t impact your output.
This is true of your entire HTML file, not just the body section. Throughout the semester, try to keep your code organized and easy to scan. It’s best practices NOT to put all of your code on one line, like this:
<body> <h1>Main Heading</h1><h2>Subheading</h2><h3>Another Slightly Smaller Subheading</h3><p>This is the paragraph section</p> </body>
Can you tell why? It’s hard to read. You can’t tell where one HTML element is closing and another one begins. 2
Formatting Your HTML
Being aware of how white space works in an HTML document is important for writing code that’s easy to read, but intending and formatting your HTML properly is equally important.
You can check out some best practices for formatting and indenting your HTML here. When your code is easy to read it makes YOUR life easier and it makes it much easier for your instructor to assess your work– and out in the “real world” writing code is often a collaborative process where team mates or clients might need to see what’s going on under the hood. Build good habits right from the beginning and make sure your code is tidy and easy to read by following these guidelines!
Your turn!
In your VS Code test document, try out the following challenges!
A) How many levels of headings (h1
, h2
, h3
, etc) can you find in HTML? Type them in test.html and see!
B) How do you create more paragraphs? Hint: you will have to repeat certain tags.
C) What happens when you add text in between these elements: <b>
type some text in here </b>
D) What happens when you add text in between these elements: <em>
and </em>
? How about <i>
and </i>
?
E) <hr/>
is an element that only has one tag rather than an opening and closing. Add it between your two paragraphs. What does it do?
When you’re done, be sure to save your file and refresh your Chrome browser window to see what you created.
If you get stuck, click page 2 for some hints!
If Chrome isn’t in the list of options to open the file with, no worries! First, make sure you have Chrome downloaded onto your computer. If you do, but it still isn’t showing up under the “Open with” options, click “Other” at the bottom of the “Open with” list and search for Chrome. Select it and click Open.↩
It will take your instructor much, much longer to help you if your code is not formatted well. ↩