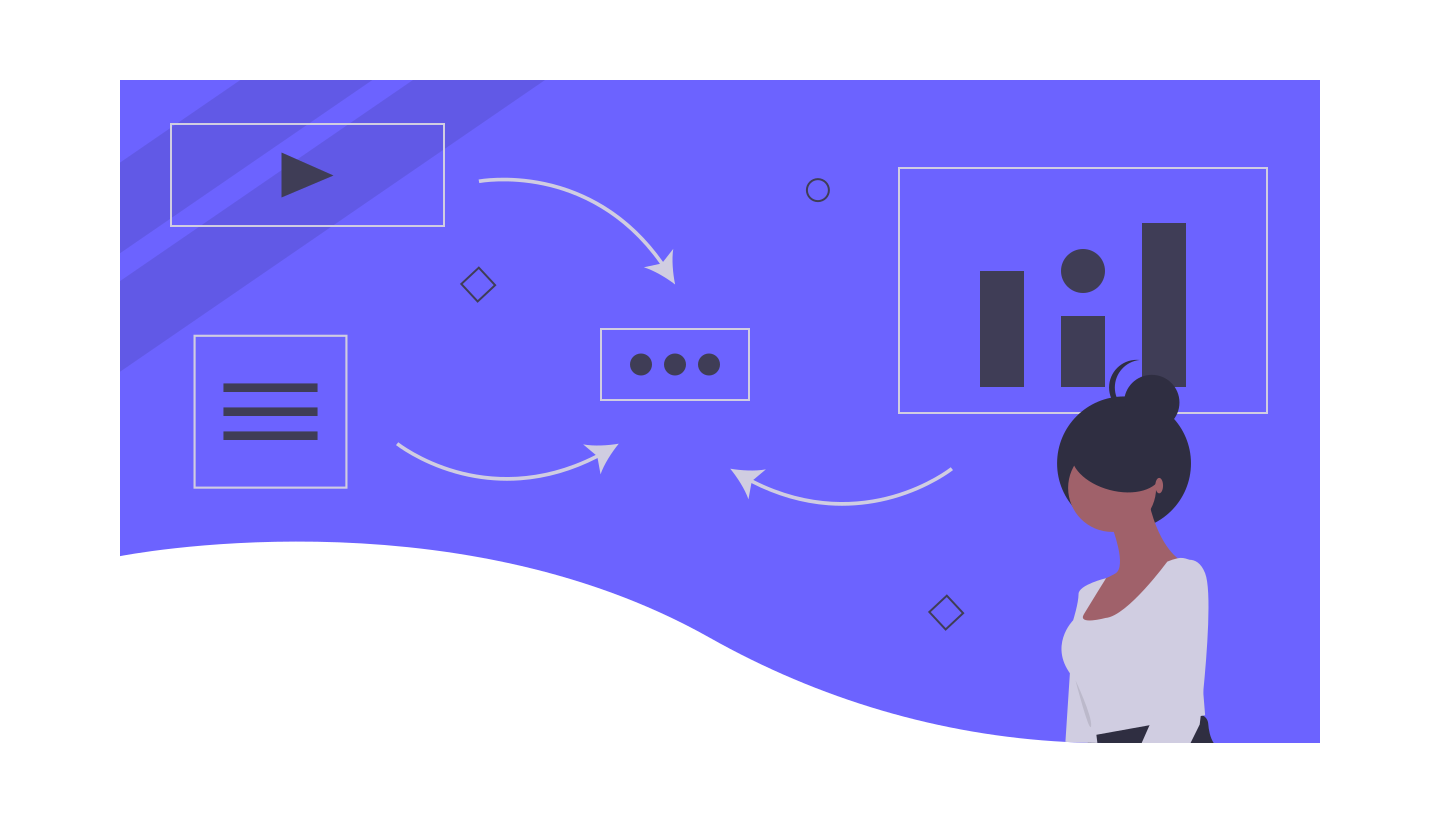
Arrays
Arrays are one of many ideas in programming that in some ways feel more complex than they actually are.
A great way to think of an array is as a box that can contain any number of items. The items in our box (array) can be any of the data types we’ve met so far—numbers, strings, etc.—but you can also put another box (array) inside of a box—this is all that’s meant by the formidable-sounding term multi-dimensional array.1
Two related concepts about arrays that are important to remember:
- The items inside your box (array) are numbered in order.
- Just like with strings, we use zero-based indexing to count the items in our array. So, to count the items in an array with three items, you’d count, “0, 1, 2—this array contains three items.”
(Oh, and if your brain gets messed up with keeping push, pop, shift, and unshift straight, don’t worry—it’s not just you. At least for me, the struggle is real, too!)
Functions
You can think of a function as a small machine you make out of code. Once you make the machine, you can use it (invoke it) repeatedly.
Let’s break down the anatomy of a simple function:
function plusThree(num) {
return num +3;
}
which we then call (invoke):
var answer = plusThree(5);
Seen altogether, we get this:
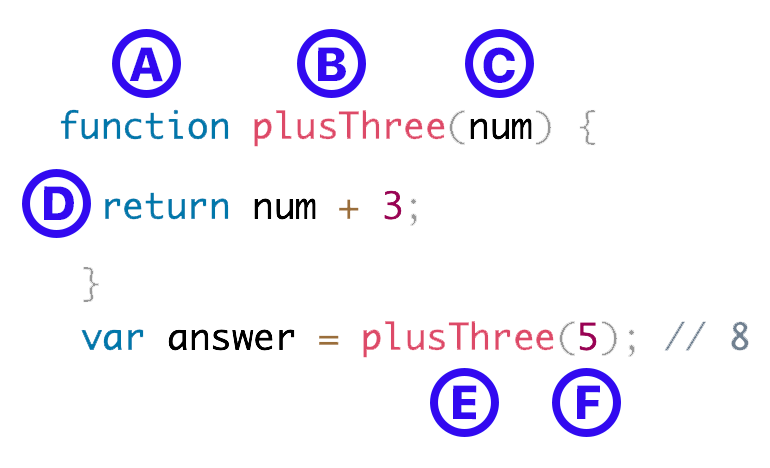
A: The function
keyword. Just like var
declares a new variable, function
declares a new function.
B: The name of our function, in this particular case, plusThree
.
C: The parameter(s) of our function. Per FreeCodeCamp, “Parameters are variables that act as placeholders for the values that are to be input to a function when it is called.” If our function is a machine, its parameters describe what types of raw materials we should put into it to get the desired output. Some functions do not contain any parameters, in which case the function name is followed by empty parentheses, like this:
function noParameters()
Opening and closing curly braces: The opening curly brace goes on the same line as the function’s declaration (as shown), and the closing curly brace goes on a separate line after all of the code contained in the function. Everything in between the opening and closing curly braces is the actual machinery of the function machine—the code that’s executed when you call the function.
D: The return statement. This defines the output of our function machine. Our plusThree
function will take a given input num
and return its value plus three.
E: Our function is called (or invoked). Our code here reads that we should assign to the newly-declared variable answer
the value returned by…
F: Passing our plusThree
function the argument 3. This is kind of a weird deal, but it’s important, so stay with me here. When we’re declaring a new function, we call the things that we want the function to take as inputs parameters (C, above). Once the function’s created, though, and we’re getting around to actually using it by calling it, we call the actual values we’re inputting in those parameter placeholder spots arguments.2
So, to pull it all together, we could say that we’ve used the function keyword to declare a function named plusThree
that accepts the parameter num
which returns the value of num+3
. We’ve then called our new plusThree
function and have passed it the argument 5
, and assigned the value returned by running our plusThree
function to the newly-declared variable answer
. Here’s the image again:
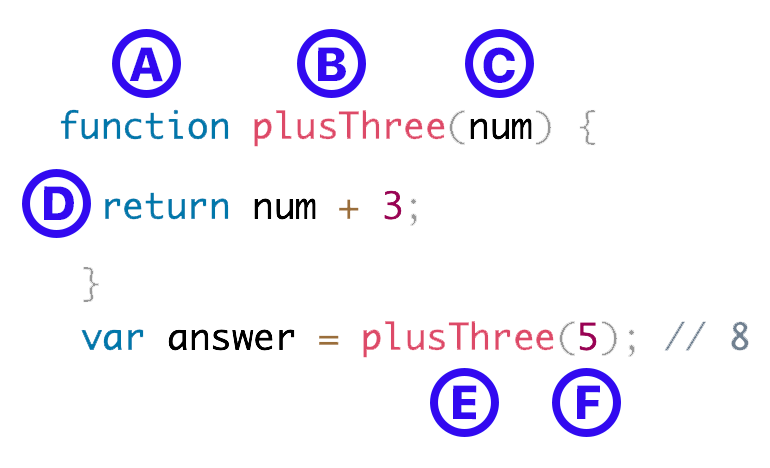
That’s a lot, but it’s worth running through in your head a number of times until you have all these terms and what they mean down pat.
Global vs. Local Scope
Scope is important, but I’ll be honest: FCC teaches us about scope at kind of an odd place. I mean, I get it: they couldn’t discuss scope earlier because we didn’t know what functions were yet, but interrupting us in the middle of learning about what a function even is is a bit much.
For the purposes of our class, we won’t get much into global vs. local scope, but it is good to know about, so here are the key takeaways (all quotes from FCC):
- “In JavaScript, scope refers to the visibility of variables. Variables which are defined outside of a function block have global scope. This means [that] they can be seen everywhere in your JavaScript code.”
- “Variables which are declared within a function (as well as the function parameters) have local scope. That means [that] they are only visible within that function.”
- “It is possible to have both local and global variables with the same name. When you do this, the local variable takes precedence over the global variable.”
And that’s it!
And of course, that box can contain yet another box, which contains yet another box, etc.—it’s turtles all the way down.↩
Why? I honestly don’t know. I tried to do some Googling, and this bit on Wikipedia was the best I could find, but I couldn’t find any history behind the difference. Let me know if you find out more!↩